10 TypeScript Tips to Improve Your Code Quality
Practical TypeScript tips that will help you write cleaner, more maintainable code.

Ege Bilge
Founder & Developer at codebiy.com
March 13, 20259 min read
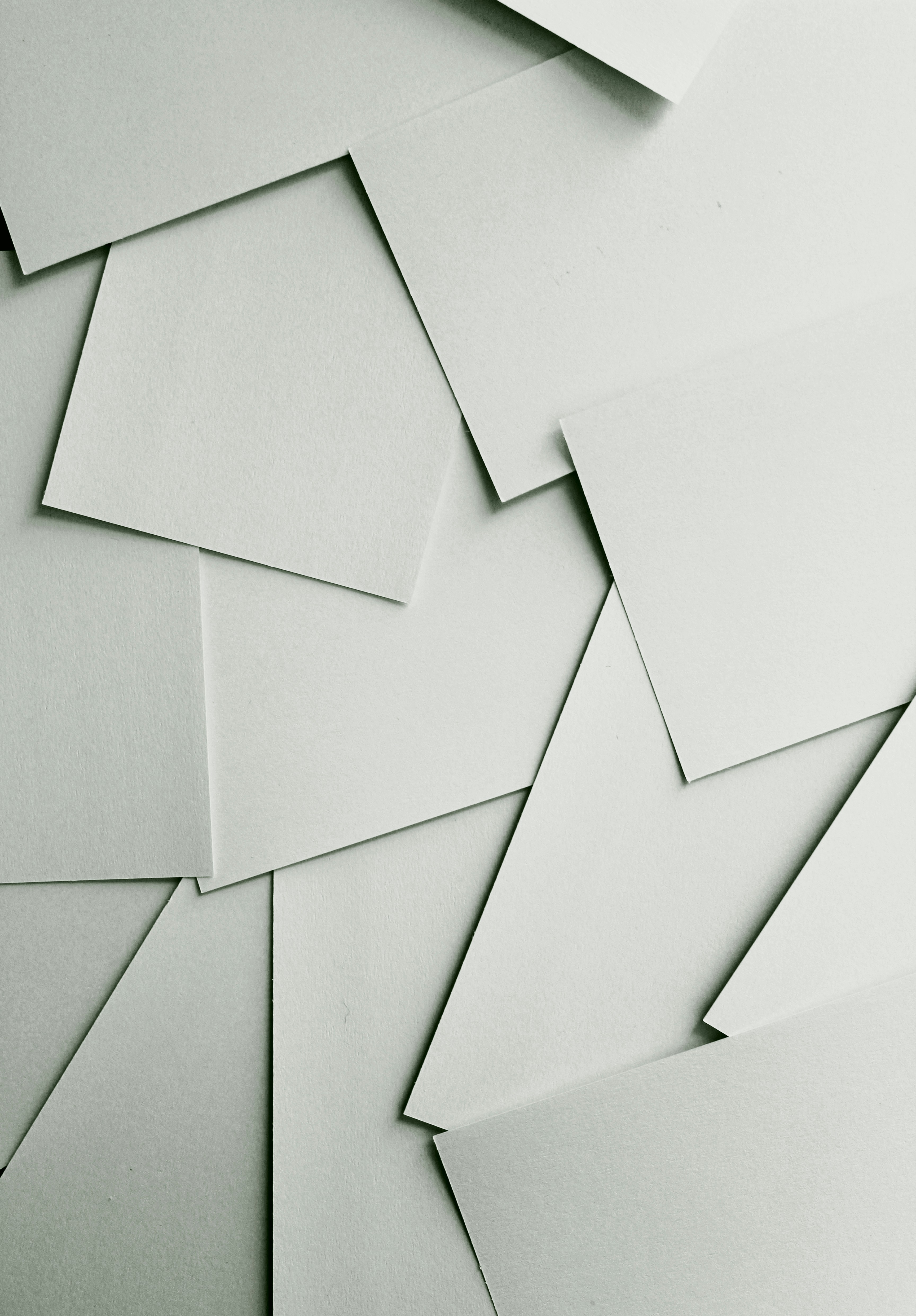
10 TypeScript Tips to Improve Your Code Quality
TypeScript has become an essential tool for building robust JavaScript applications. Here are 10 practical tips to help you write better TypeScript code.
1. Use Strict Mode
Always enable strict mode in your TypeScript configuration:
{ "compilerOptions": { "strict": true } }
This enables a range of type checking behaviors that help catch errors early.
2. Leverage Type Inference
TypeScript is smart enough to infer types in many cases. Don't add redundant type annotations:
// Good const numbers = [1, 2, 3]; // Type: number[] // Unnecessary const numbers: number[] = [1, 2, 3];
3. Use Interface for Object Types
Interfaces are more flexible than type aliases for object types because they can be extended and merged:
interface User { id: string; name: string; } interface User { email: string; // Interface merging } interface AdminUser extends User { role: 'admin'; }
4. Discriminated Unions for Complex Types
Use discriminated unions to handle different types of objects safely:
interface SuccessResponse { status: 'success'; data: any; } interface ErrorResponse { status: 'error'; message: string; } type ApiResponse = SuccessResponse | ErrorResponse; function handleResponse(response: ApiResponse) { if (response.status === 'success') { console.log(response.data); } else { console.error(response.message); } }
5. Generic Constraints
Use generic constraints to ensure type parameters meet certain requirements:
interface HasId { id: string; } function findById<T extends HasId>(items: T[], id: string): T | undefined { return items.find(item => item.id === id); }
6. const Assertions
Use const assertions for literal types:
const config = { endpoint: 'api.example.com', port: 443 } as const; // Now endpoint is type "api.example.com" instead of string
7. Exhaustive Type Checking
Ensure you handle all possible cases with exhaustive type checking:
type Shape = Circle | Square | Triangle; function getArea(shape: Shape): number { switch (shape.kind) { case 'circle': return Math.PI * shape.radius ** 2; case 'square': return shape.size ** 2; case 'triangle': return (shape.base * shape.height) / 2; default: const _exhaustiveCheck: never = shape; return _exhaustiveCheck; } }
8. Readonly Types
Use readonly modifiers to prevent accidental mutations:
interface Config { readonly apiKey: string; readonly endpoint: string; } const config: Readonly<Config> = { apiKey: 'secret', endpoint: 'api.example.com' };
9. Type Guards
Create custom type guards for complex type checking:
interface User { name: string; email: string; } function isUser(value: any): value is User { return ( typeof value === 'object' && value !== null && 'name' in value && 'email' in value ); }
10. Utility Types
Make use of TypeScript's built-in utility types:
interface User { id: string; name: string; email: string; } type UserCreateInput = Omit<User, 'id'>; type UserUpdateInput = Partial<User>; type UserReadOnly = Readonly<User>;
Conclusion
These TypeScript tips will help you write more maintainable and type-safe code. Remember that TypeScript is a tool to help you catch errors early and make your code more self-documenting. Use its features wisely, and don't overdo type annotations where they're not needed.
The key is finding the right balance between type safety and code readability. Start with these tips and adjust them based on your project's needs and your team's preferences.
Happy coding!