React Native Best Practices for 2024
Discover the latest best practices for building performant and maintainable React Native applications.

Ege Bilge
Founder & Developer at codebiy.com
March 13, 20256 min read
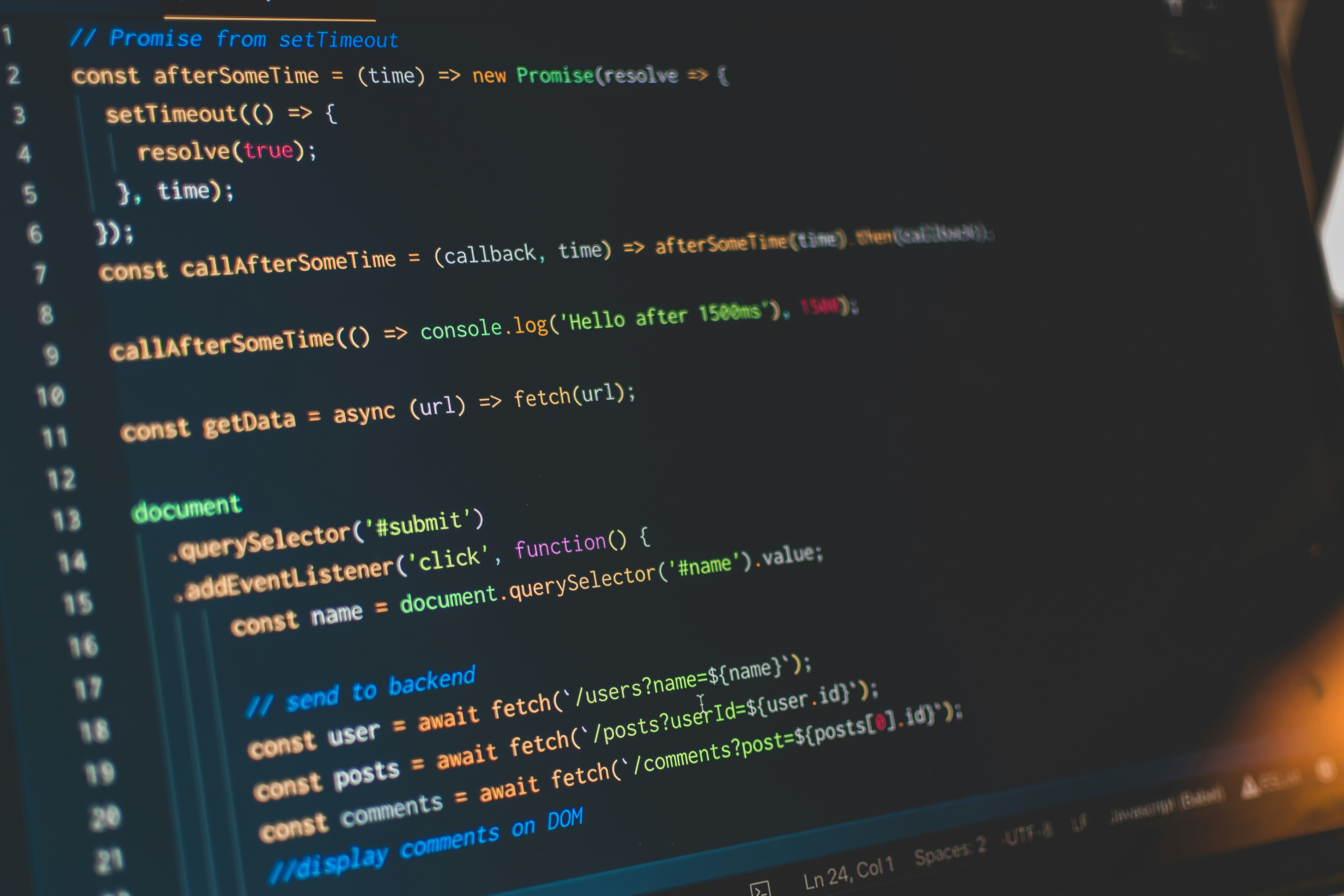
React Native Best Practices for 2024
React Native continues to be a popular choice for cross-platform mobile development. As the framework evolves, so do the best practices for building high-quality applications. In this article, we'll explore the most important React Native best practices for 2024.
Use the Latest Version
Always try to use the latest stable version of React Native. Each new version brings performance improvements, bug fixes, and new features. The React Native team has been working hard to make upgrading easier with tools like the Upgrade Helper.
Optimize Performance
Use React.memo for Pure Components
const MyComponent = React.memo(function MyComponent(props) { // Your component logic });
Virtualize Long Lists
Always use FlatList, SectionList, or FlashList for long lists instead of mapping over an array in your render method.
<FlatList data={items} renderItem={({ item }) => <Item item={item} />} keyExtractor={item => item.id} />
Avoid Anonymous Functions in Render
// Bad <Button onPress={() => handlePress()} /> // Good const handlePress = useCallback(() => { // Handle press }, [dependencies]); <Button onPress={handlePress} />
State Management
Use Context API for Simple State
For simpler applications, React's Context API can be sufficient:
const ThemeContext = React.createContext('light'); function App() { return ( <ThemeContext.Provider value="dark"> <ThemedButton /> </ThemeContext.Provider> ); }
Consider Zustand for More Complex State
Zustand provides a simple yet powerful state management solution:
import create from 'zustand'; const useStore = create((set) => ({ count: 0, increment: () => set((state) => ({ count: state.count + 1 })), }));
Navigation
React Navigation is the standard for navigation in React Native. Use the latest version and follow their recommended patterns:
import { NavigationContainer } from '@react-navigation/native'; import { createStackNavigator } from '@react-navigation/stack'; const Stack = createStackNavigator(); function App() { return ( <NavigationContainer> <Stack.Navigator> <Stack.Screen name="Home" component={HomeScreen} /> <Stack.Screen name="Details" component={DetailsScreen} /> </Stack.Navigator> </NavigationContainer> ); }
Styling
Use StyleSheet.create
Always use StyleSheet.create to define your styles:
const styles = StyleSheet.create({ container: { flex: 1, backgroundColor: '#fff', }, text: { fontSize: 16, color: '#333', }, });
Consider a Styling Library
For more complex styling needs, consider using a library like styled-components/native or restyle.
Testing
Jest for Unit Testing
Jest is the standard for testing React Native applications:
test('renders correctly', () => { const tree = renderer.create(<MyComponent />).toJSON(); expect(tree).toMatchSnapshot(); });
Detox for E2E Testing
Detox is a great choice for end-to-end testing:
describe('Example', () => { it('should show hello screen', async () => { await expect(element(by.text('Hello'))).toBeVisible(); }); });
Code Organization
Feature-Based Structure
Organize your code by feature rather than by type:
src/ features/ auth/ components/ screens/ services/ hooks/ profile/ components/ screens/ services/ hooks/ shared/ components/ hooks/ utils/
Conclusion
Following these best practices will help you build more performant, maintainable, and scalable React Native applications in 2024. Remember that best practices evolve over time, so stay updated with the latest developments in the React Native ecosystem.
Happy coding!