Getting Started with Next.js: A Comprehensive Guide
Learn how to build modern web applications with Next.js, from setup to deployment.

Ege Bilge
Founder & Developer at codebiy.com
March 13, 20258 min read
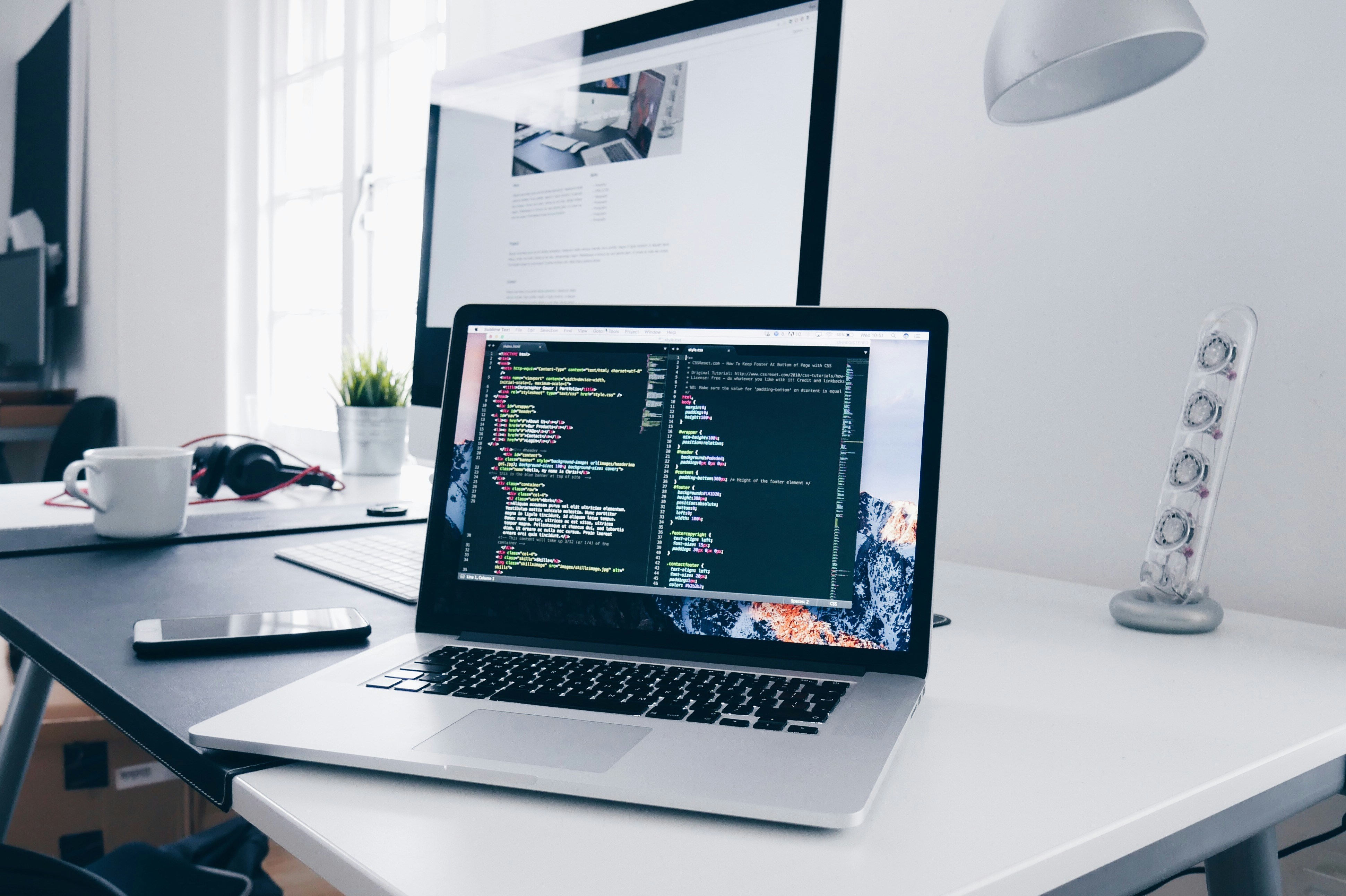
Getting Started with Next.js: A Comprehensive Guide
Next.js has become one of the most popular React frameworks for building modern web applications. It provides a powerful set of features that make it an excellent choice for both small and large-scale projects. In this guide, we'll walk through everything you need to know to get started with Next.js.
What is Next.js?
Next.js is a React framework that enables functionality such as server-side rendering, static site generation, and API routes. It's designed to provide the best developer experience with all the features you need for production.
Why Choose Next.js?
There are several reasons why Next.js has become a popular choice:
- Server-side rendering (SSR): Improves performance and SEO by rendering pages on the server
- Static site generation (SSG): Pre-renders pages at build time for even better performance
- Incremental Static Regeneration (ISR): Updates static pages after deployment without rebuilding the entire site
- API Routes: Build API endpoints as part of your Next.js application
- File-based routing: Create routes based on the file structure in your pages directory
- Built-in CSS and Sass support: Style your application with ease
- Image Optimization: Automatically optimize images for better performance
- Zero Configuration: Works out of the box with sensible defaults
Setting Up Your First Next.js Project
Let's start by creating a new Next.js project. You'll need Node.js installed on your machine.
npx create-next-app my-next-app cd my-next-app npm run dev
This will create a new Next.js project and start the development server. You can now open your browser and navigate to
http://localhost:3000
to see your application.Understanding the File Structure
Next.js uses a file-based routing system. Here's a basic overview of the file structure:
: Contains all your pages and API routespages/
: Stores static assets like images and fontspublic/
: Contains your CSS or SCSS filesstyles/
: A common place to store your React componentscomponents/
Creating Your First Page
In Next.js, each file in the
pages
directory becomes a route. For example, pages/about.js
will be accessible at /about
.Let's create a simple about page:
// pages/about.js export default function About() { return ( <div> <h1>About Us</h1> <p>This is the about page of our Next.js application.</p> </div> ); }
Data Fetching
Next.js provides several ways to fetch data for your pages:
getStaticProps
Use
getStaticProps
to fetch data at build time:export async function getStaticProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data, }, }; }
getServerSideProps
Use
getServerSideProps
to fetch data on each request:export async function getServerSideProps() { const res = await fetch('https://api.example.com/data'); const data = await res.json(); return { props: { data, }, }; }
Styling Your Application
Next.js supports various styling options:
CSS Modules
// Import the CSS module import styles from './Button.module.css'; // Use the styles export default function Button() { return ( <button className={styles.button}>Click me</button> ); }
Global CSS
// pages/_app.js import '../styles/globals.css'; function MyApp({ Component, pageProps }) { return <Component {...pageProps} />; } export default MyApp;
Deploying Your Next.js Application
Next.js applications can be deployed to various platforms. The easiest way is to use Vercel, the company behind Next.js:
- Push your code to a Git repository (GitHub, GitLab, or Bitbucket)
- Import your repository to Vercel
- Vercel will automatically detect that you're using Next.js and configure the build settings
- Click "Deploy"
Conclusion
Next.js provides a powerful and flexible framework for building modern web applications. With its built-in features like server-side rendering, static site generation, and API routes, it's an excellent choice for developers looking to build high-performance React applications.
This guide has only scratched the surface of what's possible with Next.js. As you continue your journey, explore the official documentation and community resources to learn more about advanced features and best practices.
Happy coding!