Modern Authentication Strategies for Web Applications
An overview of different authentication approaches for securing your web applications.

Ege Bilge
Founder & Developer at codebiy.com
March 13, 202510 min read
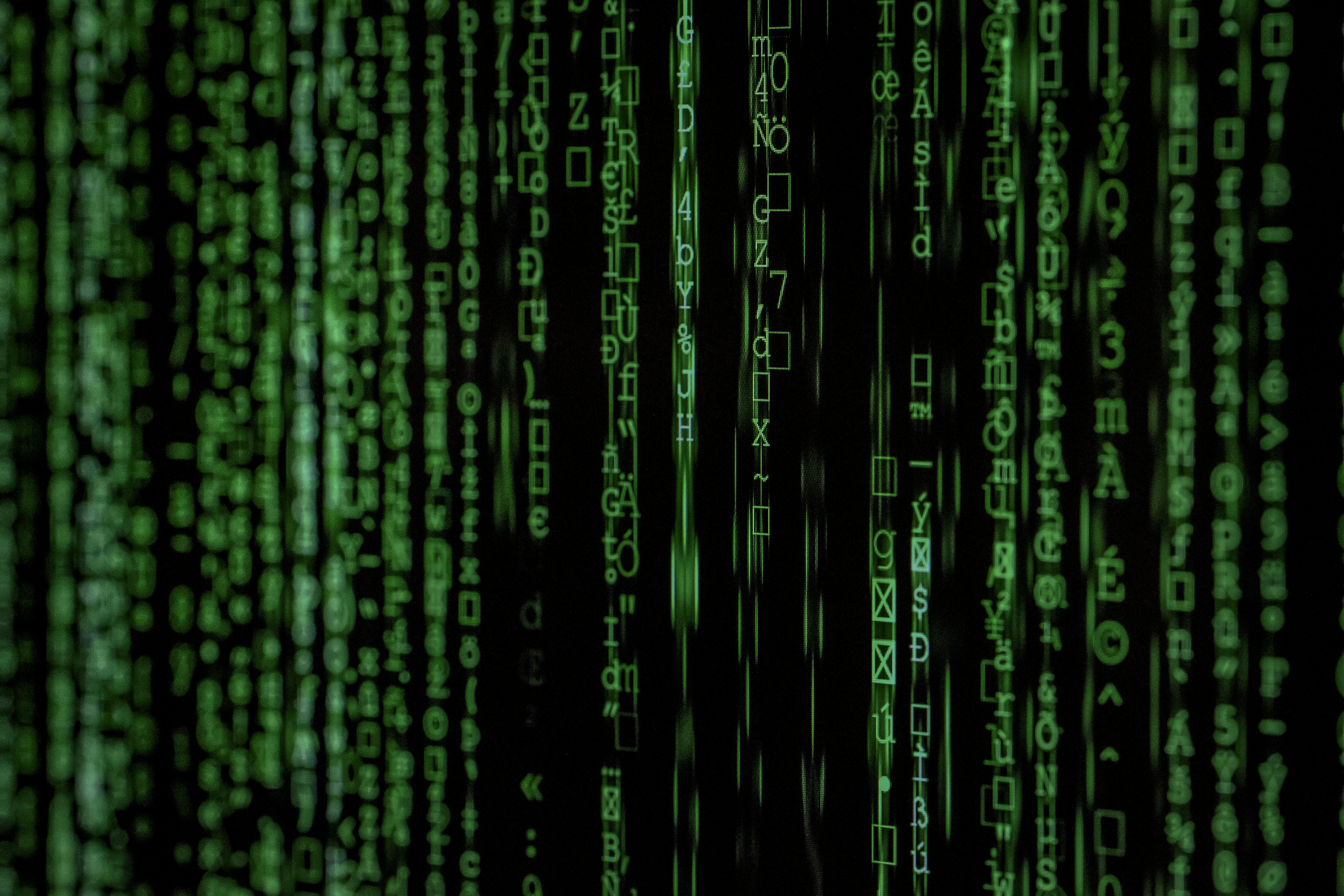
Modern Authentication Strategies for Web Applications
In today's digital landscape, securing user access to web applications is more critical than ever. Modern authentication goes beyond the traditional username and password approach, offering more secure and user-friendly options. This article explores the most effective authentication strategies for web applications in 2024.
The Evolution of Authentication
Authentication has evolved significantly from the simple password-based systems of the early web:
- Password-based authentication: The traditional approach that still forms the foundation
- Multi-factor authentication (MFA): Adding additional verification layers
- Passwordless authentication: Moving beyond passwords entirely
- Biometric authentication: Using unique physical characteristics
- Token-based authentication: Leveraging cryptographic tokens for secure sessions
Essential Authentication Patterns
1. OAuth 2.0 and OpenID Connect
OAuth 2.0 remains the industry standard for authorization, while OpenID Connect (OIDC) extends it to provide standardized authentication.
// Example of initializing Auth0 (an OIDC provider) in a React application import { Auth0Provider } from '@auth0/auth0-react'; ReactDOM.render( <Auth0Provider domain="your-domain.auth0.com" clientId="your-client-id" redirectUri={window.location.origin} > <App /> </Auth0Provider>, document.getElementById('root') );
Benefits:
- Delegated authorization
- Standardized implementation
- Widely supported across platforms
- Separate authentication concerns from application logic
2. JWT (JSON Web Tokens)
JWTs provide a compact, self-contained way to securely transmit information between parties as a JSON object.
// Example of verifying a JWT in Node.js const jwt = require('jsonwebtoken'); function authenticateToken(req, res, next) { const authHeader = req.headers['authorization']; const token = authHeader && authHeader.split(' ')[1]; if (!token) return res.sendStatus(401); jwt.verify(token, process.env.ACCESS_TOKEN_SECRET, (err, user) => { if (err) return res.sendStatus(403); req.user = user; next(); }); }
Benefits:
- Stateless authentication
- Reduced database queries
- Can contain user information and permissions
- Compatible with multiple platforms and languages
3. Passwordless Authentication
Passwordless methods eliminate the need for users to remember and manage passwords.
// Example of Magic Link implementation with Next.js API route export default async function handler(req, res) { if (req.method === 'POST') { const { email } = req.body; // Generate a unique token const token = crypto.randomBytes(32).toString('hex'); // Store token with expiration await db.tokens.create({ email, token, expires: new Date(Date.now() + 3600000) // 1 hour }); // Send email with magic link await sendEmail({ to: email, subject: 'Your login link', text: 'Click here to log in: ' + process.env.NEXT_PUBLIC_URL + '/api/auth/verify?token=' + token }); res.status(200).json({ message: 'Magic link sent' }); } }
Benefits:
- Eliminates password fatigue
- Reduces security risks from weak passwords
- Simplifies the user experience
- Potentially lowers support costs related to password resets
4. WebAuthn and FIDO2
WebAuthn represents the future of authentication, enabling passwordless biometric authentication that's both more secure and user-friendly.
// Example of registering a new credential with WebAuthn const credential = await navigator.credentials.create({ publicKey: { challenge: new Uint8Array(32), // Server-generated challenge rp: { name: "Example App", id: "example.com" }, user: { id: new Uint8Array(16), // User ID from server name: "[email protected]", displayName: "John Doe" }, pubKeyCredParams: [ { type: "public-key", alg: -7 }, // ES256 { type: "public-key", alg: -257 } // RS256 ], timeout: 60000, attestation: "direct" } });
Benefits:
- Phishing-resistant authentication
- Leverages device biometrics (fingerprint, face ID)
- Eliminates passwords entirely
- Growing browser and platform support
Multi-Factor Authentication (MFA)
MFA significantly increases security by requiring multiple forms of verification:
- Something you know: Password, PIN, security questions
- Something you have: Mobile device, security key, smart card
- Something you are: Biometrics like fingerprints or facial recognition
Implementing MFA has become significantly easier with services like:
- Auth0 Guardian
- Google Authenticator
- Microsoft Authenticator
- Duo Security
Best Practices for Modern Authentication
- Always use HTTPS to encrypt data in transit
- Implement proper session management with secure cookies and token storage
- Consider authentication as part of UX design, not just security
- Follow the principle of least privilege when assigning permissions
- Regular security audits of authentication systems
- Have a clear process for account recovery that doesn't undermine security
- Stay updated on emerging standards and vulnerabilities
Choosing the Right Strategy
The best authentication strategy depends on your specific requirements:
| Authentication Method | Security Level | User Experience | Implementation Complexity |
|----------------------|----------------|----------------|---------------------------|
| Password + MFA | High | Moderate | Low |
| Social Login | Moderate | Excellent | Low |
| Passwordless Email | Moderate-High | Good | Moderate |
| WebAuthn/FIDO2 | Very High | Excellent | High |
| JWT | Varies | Transparent | Moderate |
Conclusion
Modern web applications demand robust authentication strategies that balance security with user experience. By implementing a combination of these modern approaches, developers can significantly enhance application security while providing a seamless user experience.
As we move forward, passwordless authentication and biometric methods will likely become the standard, gradually replacing traditional password-based systems. Staying informed about emerging standards and best practices is essential for maintaining secure and user-friendly authentication systems.
Remember that authentication is just one piece of a comprehensive security strategy, and should be combined with proper authorization, data encryption, and regular security assessments.